Given a 2D matrix matrix, find the sum of the elements inside the rectangle defined by its upper left corner (row1, col1) and lower right corner (row2, col2).
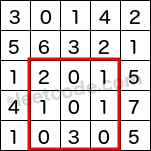
The above rectangle (with the red border) is defined by (row1, col1) = (2, 1) and (row2, col2) = (4, 3), which contains sum = 8.
Example:
Given matrix = [ [3, 0, 1, 4, 2], [5, 6, 3, 2, 1], [1, 2, 0, 1, 5], [4, 1, 0, 1, 7], [1, 0, 3, 0, 5] ] sumRegion(2, 1, 4, 3) -> 8 sumRegion(1, 1, 2, 2) -> 11 sumRegion(1, 2, 2, 4) -> 12
Note:
- You may assume that the matrix does not change.
- There are many calls to sumRegion function.
- You may assume that row1 ≤ row2 and col1 ≤ col2.
Solution:
This is the advanced version of 1D range sum.
The idea is the same, with a 2D array of summation from[0,0] to [i,j], for any range sum from [a1,b1] to [a2,b2], the range sum = sum[a2][b2] - sum[a1-1][b2]-sum[a2][b1-1]+sum[a1-1][b1-1];
I am still using an extra row and column to avoid taking care of any range starting from 0th row or 0th column.
class NumMatrix {
int[][] sum;
public NumMatrix(int[][] matrix) {
if(matrix==null || matrix.length==0 || matrix[0].length==0) return;
int m=matrix.length;
int n=matrix[0].length;
sum=new int[m+1][n+1];
for(int i=0;i<m;i++){
int res=0;
for(int j=0;j<n;j++){
res+=matrix[i][j];
sum[i+1][j+1]=res+sum[i][j+1];
}
}
}
public int sumRegion(int row1, int col1, int row2, int col2) {
return sum[row2+1][col2+1]-sum[row1][col2+1]-sum[row2+1][col1]+sum[row1][col1];
}
}
No comments:
Post a Comment